Cross Site Scripting (XSS)
ColdFusion Security Guide
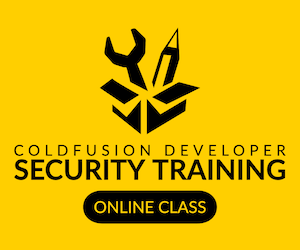
The Risk
Cross Site Scripting attacks allow an attacker to run arbitrary client side scripts (such as javascript) on your web application.
The level of risk to your application depends on the sensitivity of the operations that users can perform.
Vulnerable XSS Code Example
<cfoutput>Hi #url.name#</cfoutput>
In this ColdFusion XSS example we are simply outputting the variable url.name
. This variable comes from the URL query string and is there for untrusted.
Examples of Untrusted Variables
URL
- variables in the url scope.FORM
- variables in the form scope.CGI
- variables in the CGI scope many of which are derived from the HTTP request.COOKIE
- variables in the cookie scope.- HTTP Headers
- The entire HTTP Request is untrusted
The definition of an untrusted variable may vary from organization to organization and the level of security required by an application. For example most applications will treat variables coming from a database table as untrusted and encode them. It is a good practice to error on the side of caution if you are unsure if a variable can be trusted. This also makes a code security review easier because you don't have to stop and check if the variable is trusted or not.
Mitigating XSS in ColdFusion and Lucee
To prevent XSS you can either strip unsafe characters, or encode them. The problem with stripping unsafe characters is that it can be difficult to know which characters are unsafe. If you are stripping all characters except the digits 0-9 then you can be pretty certain that it is a safe mitigation.
Both Adobe ColdFusion and Lucee provide encoder functions designed for mitigating cross site scripting. The functions are:
encodeForHTML()
encodeForHTMLAttribute()
encodeForCSS()
encodeForJavaScript()
encodeForURL()
It is important to use the correct encoder function for the correct context.
Example XSS Mitigation with encodeForHTML
You would use the encodeForHTML function within the innerHTML of a HTML element. For example inside of a div, or the inner text of an anchor tag:
<cfoutput><p>Hi #encodeForHTML(url.name)#</p></cfoutput>
You should not use the encodeForHTML inside the attribute values of a HTML tag, there is another function for that called encodeForHTMLAttribute
which we will look at next:
Example XSS Mitigation with encodeForHTMLAttribute
When you are outputting a ColdFusion variable inside of a HTML attribute you would use the encodeForHTMLAttribute
tag.
<cfoutput><input value="#encodeForHTML(form.name)#"></p></cfoutput>
Example XSS Mitigation with encodeForCSS
ColdFusion variables found inside of CSS should be encoded with the encodeForCSS
function.
<style>body { color: #encodeForCSS(url.color)#;}</style>
This also includes the style
attribute of HTML tags:
<div style="color: #encodeForCSS(url.color)#">yup</div>
Example XSS Mitigation with encodeForJavaScript
ColdFusion variables that occur inside of Java Script should be encoded with the encodeForJavaScript
function.
<script>var id = #encodeForJavaScript(url.id)#;</script>
This also includes all java script event handlers in HTML tags:
<button onclick="go(#encodeForJavaScript(url.id)#)">go</a>
And finally all javascript:
URIs:
<a href="javascript:go(#encodeForJavaScript(url.id)#)">go</a>
Example XSS Mitigation with encodeForURL
When outputting a ColdFusion variable within the URL use the encodeForURL
function.
<a href="page.cfm?id=#encodeForURL(url.id)#">go</a>
Using the encodefor
attribute of cfoutput
The cfoutput
tag also has an encodefor
attribute which accepts the values html
, htmlattribute
, css
, javascript
or url
.
This is a nice shortcut if you have a bunch of variables to encode in the same cfoutput tag:
<cfoutput encodefor="html">Hi #url.name#, #url.message#</cfoutput>
Fixinator
Fixinator is a CFML source code security scanner that can find and fix several types of security issues, including XSS.
Learn More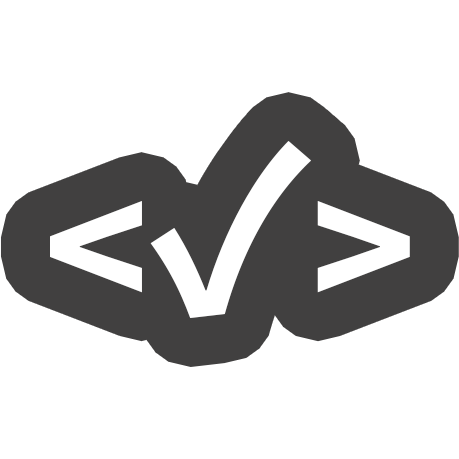
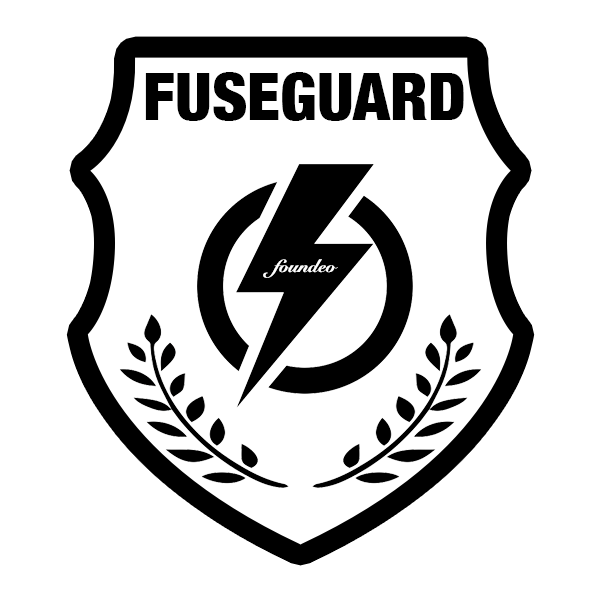
FuseGuard
FuseGuard is a web application firewall can runs onRequestStart to block or log malicious requests to your ColdFusion web applications. FuseGuard has a cross site scripting filter that can block some XSS attacks against your site before they hit your application code.
Learn More